Android Studio Sample Code
The ArcGIS Runtime SDK for Android samples are tools for developers to. In the repo's README file to work with the sample projects in Android Studio. One of the best ways to learn about Android is to checkout Android code in the wild. The following is good Android sample code to review: We need help from the broader community to improve these guides, add new topics and keep the topics up-to-date. See our contribution guidelines here and our topic.
Getting started The finished game will allow the player to move left and right by holding the corresponding side of the screen near the bottom. As the player also needs to shoot at the invaders we will respond to when the screen is touched slightly higher than the lower part as well. The Invaders will slide across the screen flapping their arms and spitting bullets. If they hit a player he loses a life if they hit a shelter it will crumble until eventually the player has nowhere to hide. When the invaders reach the side of the screen they will drop down and scroll back the other way; a little bit faster. Eventually they will be moving so fast the player is doomed.
The space invaders must be taken out quickly and efficiently to survive. To get started create a new project in Android Studio, call it Space Invaders and name the Activity SpaceInvadersActivity then read on because we will do things slightly differently compared to the Breakout project. Making the game full-screen landscape We want to use every pixel that the device has to offer so we will make changes to the app’s AndroidManifest.xml configuration file. In the project explorer pane in Android Studio double click on the manifests folder, this will open up the AndroidManifest.xml file in the code editor. In the AndroidManifest.xml file, locate the following line of code, android: name = '.SpaceInvadersActivity'. Place the cursor before the closing shown above.
Tap the enter key a couple of times to move the a couple of lines below the rest of the line shown above. Immediately below SpaceInvadersActivity but BEFORE the newly positioned type or copy and paste these two lines to make the game run full screen and lock it in the landscape orientation.
Android:theme='@android:style/Theme.NoTitleBar.Fullscreen' android:screenOrientation='landscape' Let’s talk a bit about the assets we will use in Space Invaders. Click to download the bonus!
Also, note that all the bonus downloads for this and every future tutorial is available on an exclusive download area for my Patreon subscribers. The Graphics In this project, we will use a mixture of bitmaps from external graphics files for the invaders and player ship and use the Canvas class’ drawRect method to represent the lasers and the shelters.
Something worth mentioning at this point is that even the classes that use bitmaps will still have a RectF object because although we don’t need it for drawing we can still use it for collision detection. More on this later in the project. Before we get coding we want to add the player ship graphic and two different graphics for the two frames of animation for our invader to our project files so we can use them in our code. The graphics are white so they are displayed below on a blue background. Here they are. The second frame of animation for the invader You can download them by right-clicking on the images then select the Save image as option. Just make sure the file names are kept the same.
They are named playership.png, invader1.png and invader2.png. Add the graphics to the drawable folder within your Android Studio folders. The sound We will have a menacing two-tone sound to represent the invader’s movement. This sound will speed up as the invaders speed up. I have called these two sounds “uh” and “oh” because if we were attacked by invaders we might say, “uh oh”. We also need sounds for shooting a laser, the player being hit, an invader being hit and a shelter being damaged.
Of course, we need to add the sound files to our project. You can create your own or download mine by right-clicking on the files listed below. Just make sure you use exactly the same file names.
You can also listen to each of the sounds by using the media controls below each of the links. When you have your preferred sound FX, using your operating system’s file browser go to the app src main folder of the project and create a folder called assets. Add your sound file to this folder. The class structure For this project, we will vary slightly from the structure of the Breakout project.
But far from adding complexity, we will see how by separating out further the code in the different files we will make our Space Invaders project simpler to write, manage and extend. Each game object will be represented by its own class. So we will have one for the player’s ship, one for an invader, a bullet and the brick of a defence shelter. Furthermore, we will also take the inner class which represents the view of the game and make it a class in its own right. It will be called SpaceInvadersView. This changes nothing about how we code it internally but it does make the whole project more manageable.
We want to create classes to represent the view and the game objects we have just discussed. We will require the following classes.
SpaceInvadersView, Invader, PlayerShip, DefenceBrick and Bullet. By creating empty implementations of all these classes we can then declare objects of them in SpaceInvadersView right away. This avoids constantly revisiting SpaceInvadersView to add new declarations. All we will need to do is implement each object, in turn, initialize it, update it and draw it. With this in mind let’s create those empty classes.
Now, Right-click the java folder in the Android Studio project explorer as shown in the next image. The completed Space Invaders game running on an Android phone Space Invaders final thoughts The next project will have a scrolling world. That is the entire game area will not be drawn to the screen at one time. This will introduce the concept of a viewport which defines what the player can see at any given frame of play. We can also use the viewport to clip objects that don’t need updating or collision checking which in turn makes our code faster. There is also a project you might be interested in to introduce a so we can have multiple objects all doing their own cool animations; like the Space Invaders arm waving but with an unlimited and a dynamic number of frames. Please leave any comments below.
Thanks and happy coding. Hi Deeps, I don’t have the code to hand at the moment (other than the website). To test things out I just created a new project as described, created the six classes, pasted the code for SpaceInvadersActivity. I get errors in exactly the same places that you describe, but copy and pasting the code for SpaceInavdersView fixes this. Now however I have errors in SpaceInvadersView but adding the other classes, graphics and sound fixes this. You will have errors throughout the project but if you follow the steps in order it should work out in the end. If not, let me know the specific errors and I will try and help you troubleshoot.
Good luck,. Hi Deeps, It could be that in the project configuration there is a version where you don’t have the build tools (api etc.) installed. But I would try this first: 1) Create a new project in Android Studio, call it Space Invaders and name the Activity SpaceInvadersActivity (as before) then make sure you leave all other settings at the default suggested by Android Studio especially the Android sdk versions.
2) Before you add/remove any code at all see if this”Hello world!” project will build and run. 3a) If it does run copy/paste all the java/image/sound files from your previous project and try to run again.
Don’t copy paste the AndroidManifest file as this will contain configuration info which might be part of the problem. 3b) If it doesn’t run refer to the link below to try and fix the build version problem by following the info at this link as a rough guide. Good luck Deeps. Hi Deeps, life is manic lately. I am on the go writing on a laptop much of the time. My father was taken ill on Monday, my partner is away at a conference for two weeks, the kids are due back at school tomorrow and I really can’t stop to do calls etc.
I do get a couple of minutes every now and then to reply to comments however and if you tell me what exactly didn’t work I might be able to suggest something else. Did the new “Hello world!” project run?
If it didn’t I would try making sure you have latest api installed. Tools Android SDK Manager. Get the Hello world project to run and then add the Space Invaders code and assets to it. Pc oyun indir.
Sorry I can’t chat at the moment. I really want you to be able to get this running I just can’t commit to face to face/chat at the moment. All the best, John. Hi there a11n, As the variables seem to be changing this is a bit odd.
The only thing I can think of suggesting is first that the controls are very basic and if you hold down both sides of the screen simultaneously the code won’t handle it properly and you might get unexpected results. The other thing which can make the input handling less responsive is if you haven’t implemented the “making the game full screen” near the start of the tutorial as this would leave hidden part of the control area at the bottom of the screen. Let me know how you get on and if it still doesn’t work are you able to give any more details at all and I will try and help you solve this. It is full screen.
I add after playerShip.setMovementState(playerShip.RIGHT); the code System.out.println(“1″ + motionEvent + “2 ” + (screenX / 2)); System.out.println(“2″); and after playerShip.setMovementState(playerShip.LEFT); yhe code System.out.println(“1″ + motionEvent + “2 ” + (screenX / 2)); System.out.println(“1″); to see if my program enter in the first case of the “if” and when i press in the right place of the screen in my console is diplayed “2”, same for the left place of the screen.This what i’ve done. Hi Adi, The most likely solutions are either that the PlayerShip class is in a different folder to the other classes or the class itself has been misspelt. It is quite easy in Android Studio to create a new class in the Test module instead of the main package folder. If you look in the project explorer window (where the files and folders are shown on the left), you can expand the Java folder and there will be two options for placing new classes.
All your Java files should be in the top one? Not the (androidTest) one. Thank you very much John for the tutorial. It was excellent.
I have a couple of general questions. With the finish product on a Nexus 7 tablet, the player moves around “jerkily”. I’m able to move and fire, etc. But the movement of the player is jerky. I realize this is a beginning demo, but could you give some general ideas on how you would make the action smoother?
Is this where OpenGL comes in? If I understand this demo fairly well, which of your books would you recommend? You have at least 3 dealing with Android and Games. Perhaps one is more up to date? And I believe I read somewhere on your blog a comment from you along the lines of when during the year the books are discounted or possibly a discount code. Then again, possibly it’s wishful thinking on my part!
Thanks, Dave. Hi Andrew, thanks for the message. Space invaders should be reasonably smooth moving although the simplistic controls might make things a bit eratic. If the game runs slow try the version in my free android app to see if that has the same issues on your device.
Vb Sample Code Visual Basic
Anrdroid programming for beginners is non-games so not suitable if games is your thing. Learning java building android games is for complete java novices so not for you if you are comfortable with classees, threads, basic java. Android game programming by example has 3 projects in it. The first is about the same level as space invaders, the second is a platformer with an explorable scrolling world and the third an asteroids game using opengl es2. This one might be most appropriate. You can read about them on the books tab and the publishers site linked to from there and you can play the games that are built on the free android app.
See the tab at the top of the page. Packt do have discounts on and off thoughout the year but I am not sure when the next is. Hope this helps. John, I was reviewing the code with an aim to making minor modifications. I realized I have no idea what you are doing with: private Thread gameThread = null; As far as I can tell, you’re just starting the thread, and joining the thread. I come from a Windows background (C#/C). I was expecting to see a thread procedure.
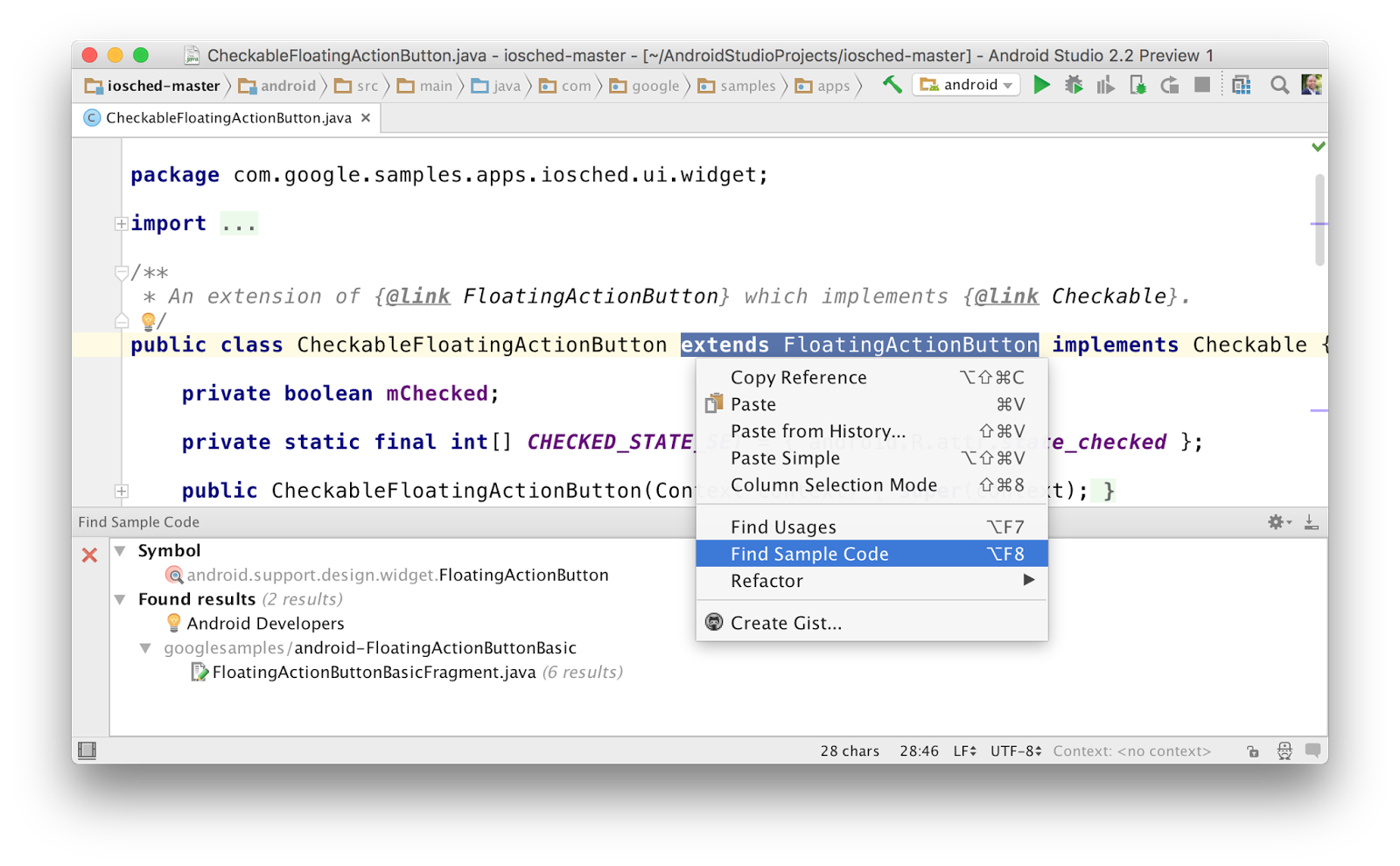
That is a function that does work on this new thread. I don’t see any of that. It’s as if the thread is doing nothing. All the works seems to be on the main GUI thread.
Is this some Android trick? Perhaps you could point me to somewhere on your site or elsewhere that explains it? Thanks,.If you play the game and click to the left of the player ship, the ship will go left.
That’s correct. However, once the ship gets over to the left of the screen, if you click to the right of the ship, but still on the left hand side of the ship, the ship still goes left. I changed code to take into account whether the click is the left or right of the ship. It seems better (to me) now. Another improvement I’ll try and make is to “grab” the ship and drag it to the left or right. I’m hoping you post a “scrolling game” soon.
Perhaps Defender? All you need to do is draw a bitmap the size of the whole screen and then draw all the other objects on top of it. This code is untested, but it goes something like this: // Create a bitmap outside the game loop Bitmap background = BitmapFactory.decodeResource(getResources,R.drawable.background); // Each time, in the draw method of the game loop // Draw the background first canvas.drawBitmap(background, paint); // Don’t fill the screen with color after or you will hide it // Draw all the other objects To change object colors just use the next line of code before the object you want to change the color of. // Choose the brush color for drawing // The numbers are 255 (full opacity) 255, 255, 255(full red, green and blue) // The result is white mPaint.setColor(Color.argb(255, 255, 255, 255)); Hope this helps. Hi Lance, nice to hear from you. All you need to do is draw a bitmap the size of the whole screen and then draw all the other objects on top of it. This code is untested, but it goes something like this: // Create a bitmap outside the game loop Bitmap background = BitmapFactory.decodeResource(getResources,R.drawable.background); // Each time, in the draw method of the game loop // Draw the background first canvas.drawBitmap(background, paint); // Don’t fill the screen with color after or you will hide it // Draw all the other objects Hope this helps.
I’ve tried following this tutorial but either I have very little understanding of what is going on or my software is faulty I followed the steps exactly, but I get a red line for: setContentView(spaceInvadersView); in SpaceInvadersActivity.java also for: bitmap1 = BitmapFactory.decodeResource(context.getResources, R.drawable.invader1); in Invader.java and PlayerShip.java I get ” cannot resolve symbol ‘R’ ” for SpaceInvadersView spaceInvadersView; in SpaceInvadersActivity.java it doesn’t seem to find SpaceInvadersView, no matter what I do Please help. Hi Mike, Here are some suggestions of likely causes.
“I get a red line for: setContentView(spaceInvadersView);”. Most likely the class, is misnamed, has an error or is in a different folder (left hand side project explorer.), to the other classes. ” cannot resolve symbol ‘R’ ” Is usually be fixed by syncining the project. Try Tools Android Sync project with Gradle files. “it doesn’t seem to find SpaceInvadersView”. I am not sure what you mean here. Can you explain it to me another way.
Java Sample Code
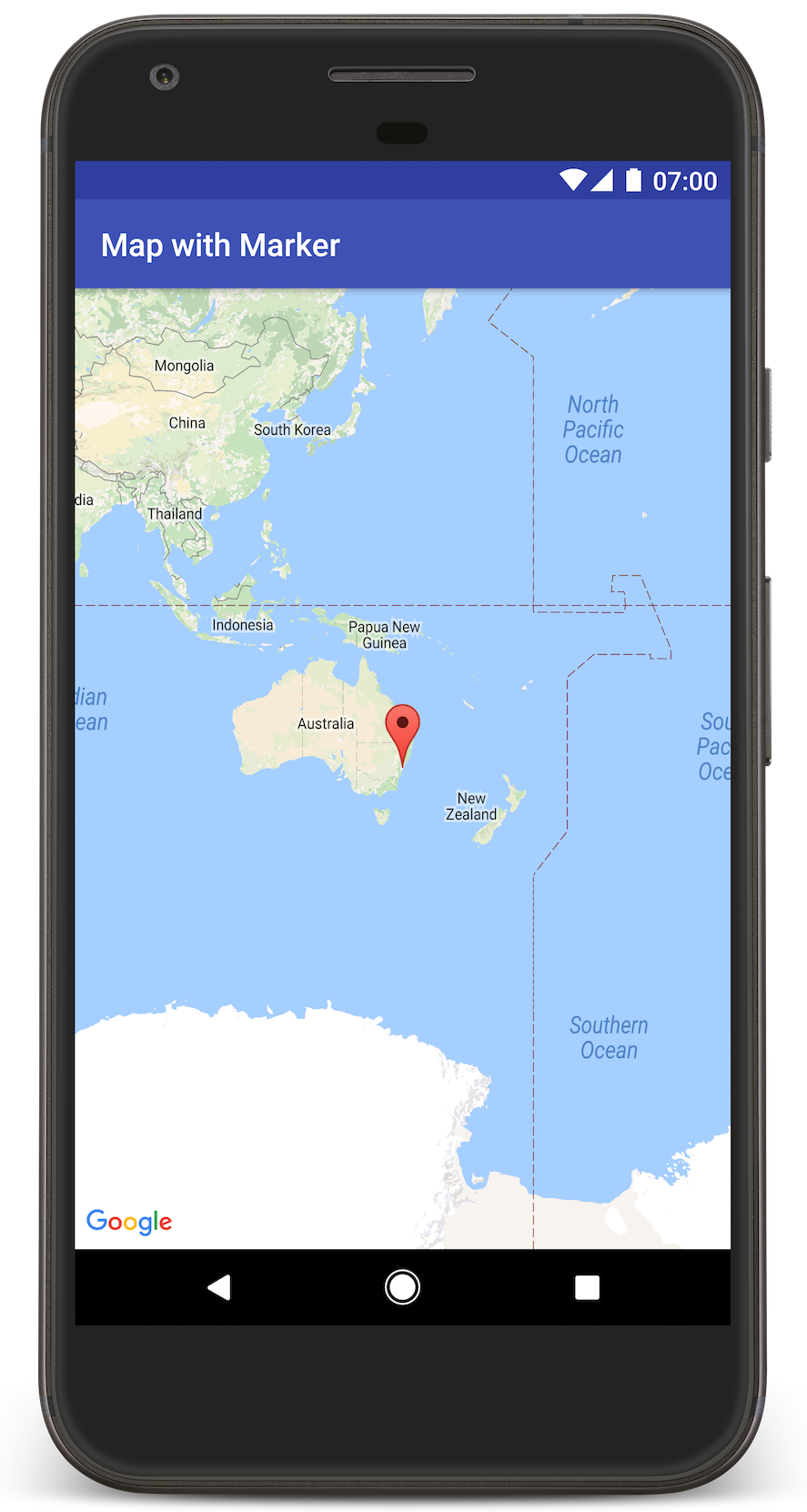
I hope this helps. Let me know either way and I will try and help you get it working. Hi again John, I managed to code the previous code that I had trouble on. But I do have another question for you if you don’t mind. I am having trouble with a GAME OVER screen.
I know I have to call it to the surface view but no idea how to go about this. For instance a brand new view where the layout background is black, text is GAME OVER.
I have no idea on how to add this to the Surface View. Any tips on how I am able to do so? ( I made sure to /find this post, just in case someone had ask so already). There are a few ways to do this. The quickest, without changing the structure of the code, is to introduce some game states. This can be done with some simple Boolean variables perhaps ( homeScreen, hiScoreScreen, gameScreen) but could be more neatly done with an enumeration.
Download suara sirine polisi. You could then just wrap different parts of the drawing and input handling code in if statements to represent what screen should currently be shown. So when the player loses his last life you could set homeScreen = true. Then the draw and input handling parts of the code would respond differently. Hope this helps a bit.
I'm new to Android and Java.
I'm using the latest Android Studio on my windows 10.
I simply created an empty activity and pasted the code from
to my MainActivity.
Following its comments, I also added uses-permission in my manifest.
I've read all Q&As, I believe the manifest is good.
However I keep getting setAudioSource failed with the callstack in the end of post.
The error is the same on both my Nexus 5 and my Nexus 5 Virtual device.
Could you help me, please? Thanks!
My code is below:
Output in Android Monitor Verbose mode:
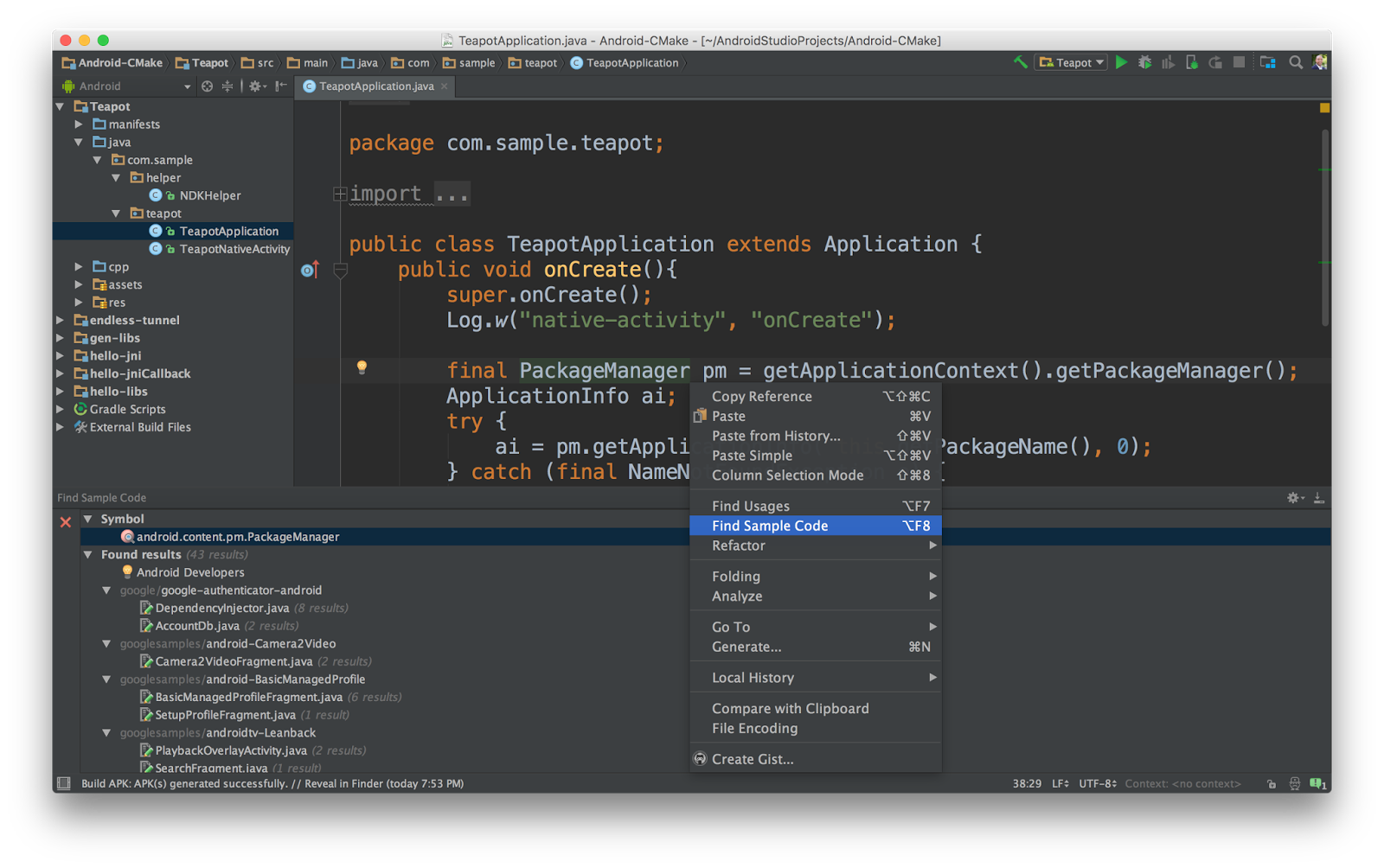
2 Answers
Yes there is! You can call requestPermissions() from your Activity or Fragment. This example fits for a call from an Activity
Dec 2, 2018 - Jiskha Homework Help. English rules 2. English rules 2 anwers - Sheet 6. I was searching around and cannot find the rules for the forum. Reset Epson Adjustment Program R290. Welcome visitor please English Rules! Online English Rules! English rules 2 sheet 1 answers? Any tips for answering. English rules 2 homework program answers sheet 1477 iv. How to use the program; About Us; Online Publications. English Rules! 1; English Rules! 2; English Rules! 3; For Schools; Contact Us. Sheet 4 - Revision. WWP-80202 - English Rules! 2 Student Book 2nd. Tear out sheet provides instruction. Is part of our full range of english homework programs for use in.
Then you can check in onRequestPermissionResult if permission was granted:
For good practice, you should check if a permission was already granted (to avoid annoying the user by asking every time.)
Finally found i need to go to Setting->App->Permission-> slide on the Microphone and Storage manually. Why didn't I finding anything mentioning this.
Is there a way to explicitly prompt the user to give these permissions, instead of silently crashing with the settings hidden so deep in the phone??